Sockets and the socket API are used to send messages across a network. They provide a form of inter-process communication (IPC). The network can be a logical, local network to the computer, or one that’s physically connected to an external network, with its own connections to other networks. The obvious example is the Internet, which […]
Category: Data Analytics
Python Code Quality: Tools & Best Practices
In this article, we’ll identify high-quality Python code and show you how to improve the quality of your own code. We’ll analyze and compare tools you can use to take your code to the next level. Whether you’ve been using Python for a while, or just beginning, you can benefit from the practices and tools […]
Documenting Python Code: A Complete Guide
Welcome to your complete guide to documenting Python code. Whether you’re documenting a small script or a large project, whether you’re a beginner or seasoned Pythonista, this guide will cover everything you need to know. We’ve broken up this tutorial into four major sections: Why Documenting Your Code Is So Important: An introduction to documentation […]

Fast, Flexible, Easy and Intuitive: How to Speed Up Your Pandas Projects
If you work with big data sets, you probably remember the “aha” moment along your Python journey when you discovered the Pandas library. Pandas is a game-changer for data science and analytics, particularly if you came to Python because you were searching for something more powerful than Excel and VBA. So what is it about […]
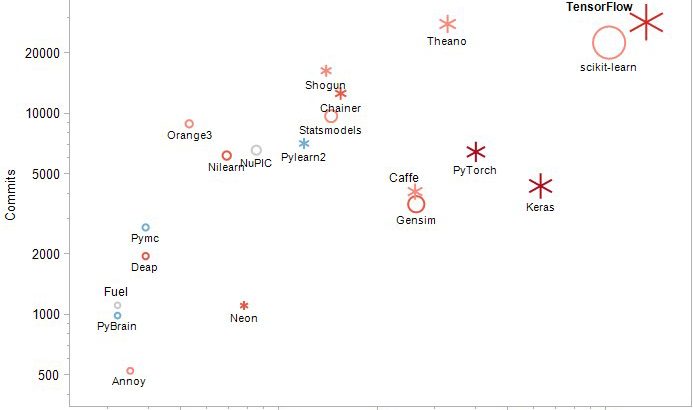
Top 20 Python AI and Machine Learning Open Source Projects
Getting into Machine Learning and AI is not an easy task. Many aspiring professionals and enthusiasts find it hard to establish a proper path into the field, given the enormous amount of resources available today. The field is evolving constantly and it is crucial that we keep up with the pace of this rapid development. […]
Lists and Tuples in Python
Lists and tuples are arguably Python’s most versatile, useful data types. You will find them in virtually every nontrivial Python program. Here’s what you’ll learn in this tutorial: You’ll cover the important characteristics of lists and tuples. You’ll learn how to define them and how to manipulate them. When you’re finished, you should have a […]

Basic Statistics in Python: Probability
When studying statistics, you will inevitably have to learn about probability. It is easy lose yourself in the formulas and theory behind probability, but it has essential uses in both working and daily life. We’ve previously discussed some basic concepts in descriptive statistics; now we’ll explore how statistics relates to probability. Prerequisites: Similar to the […]
Pipenv: promises a lot, delivers very little
Pipenv is a Python packaging tool that does one thing reasonably well — application dependency management. However, it is also plagued by issues, limitations and a break-neck development process. In the past, Pipenv’s promotional material was highly misleading as to its purpose and backers. In this post, I will explore the problems with Pipenv. Was […]
Reading and Writing CSV Files in Python
Let’s face it: you need to get information into and out of your programs through more than just the keyboard and console. Exchanging information through text files is a common way to share info between programs. One of the most popular formats for exchanging data is the CSV format. But how do you use it? […]

Generating Random Data in Python (Guide)
How random is random? This is a weird question to ask, but it is one of paramount importance in cases where information security is concerned. Whenever you’re generating random data, strings, or numbers in Python, it’s a good idea to have at least a rough idea of how that data was generated. Here, you’ll cover […]
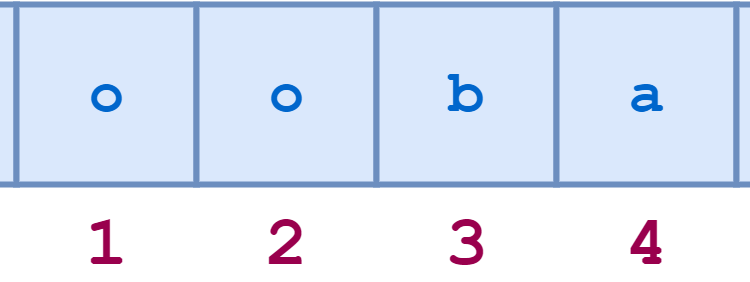
Strings and Character Data in Python
In the tutorial on Basic Data Types in Python, you learned how to define strings: objects that contain sequences of character data. Processing character data is integral to programming. It is a rare application that doesn’t need to manipulate strings at least to some extent. Here’s what you’ll learn in this tutorial: Python provides a […]
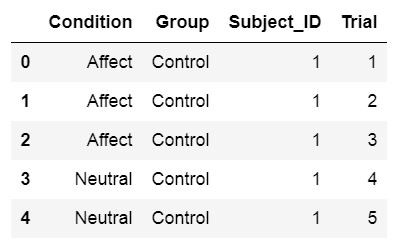
A Basic Pandas Dataframe Tutorial for Beginners
In this Pandas tutorial we will learn how to work with Pandas dataframes. More specifically, we will learn how to read and write Excel (i.e., xlsx) and CSV files using Pandas. We will also learn how to add a column to Pandas dataframe object, and how to remove a column. Finally, we will also learn […]
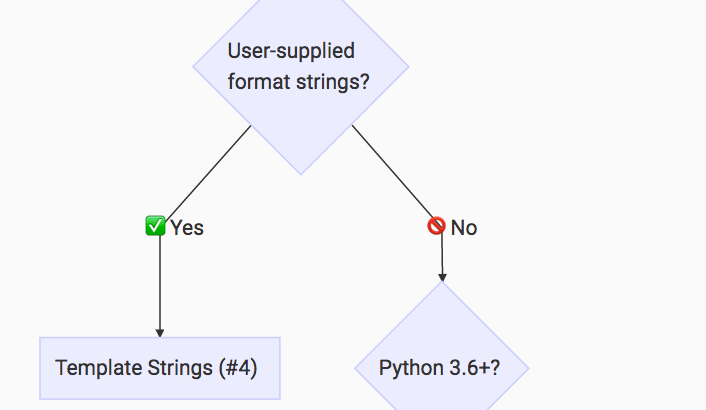
Python String Formatting Tutorial
Remember the Zen of Python and how there should be “one obvious way to do something in Python”? You might scratch your head when you find out that there are four major ways to do string formatting in Python. In this tutorial, you’ll learn the four main approaches to string formatting in Python, as well […]
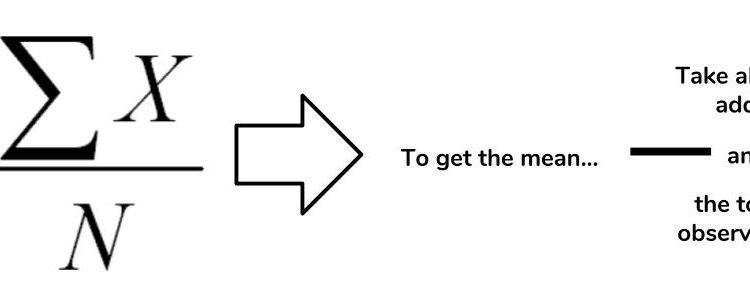
Basic Statistics in Python: Descriptive Statistics
The field of statistics is often misunderstood, but it plays an essential role in our everyday lives. Statistics, done correctly, allows us to extract knowledge from the vague, complex, and difficult real world. Wielded incorrectly, statistics can be used to harm and mislead. A clear understanding of statistics and the meanings of various statistical measures […]
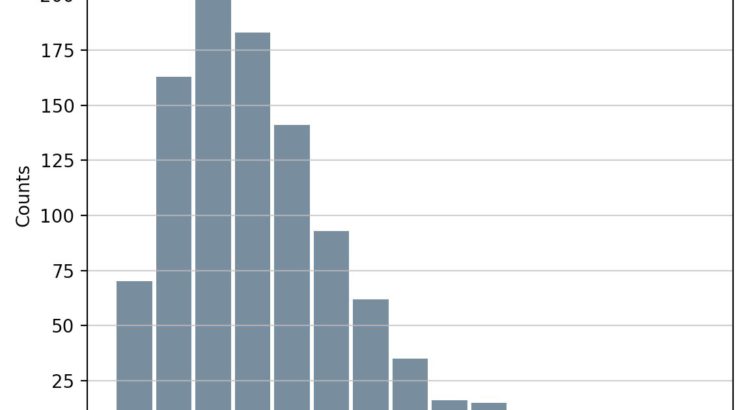
Python Histogram Plotting: NumPy, Matplotlib, Pandas & Seaborn
In this tutorial, you’ll be equipped to make production-quality, presentation-ready Python histogram plots with a range of choices and features. If you have introductory to intermediate knowledge in Python and statistics, you can use this article as a one-stop shop for building and plotting histograms in Python using libraries from its scientific stack, including NumPy, […]